Logic Flow
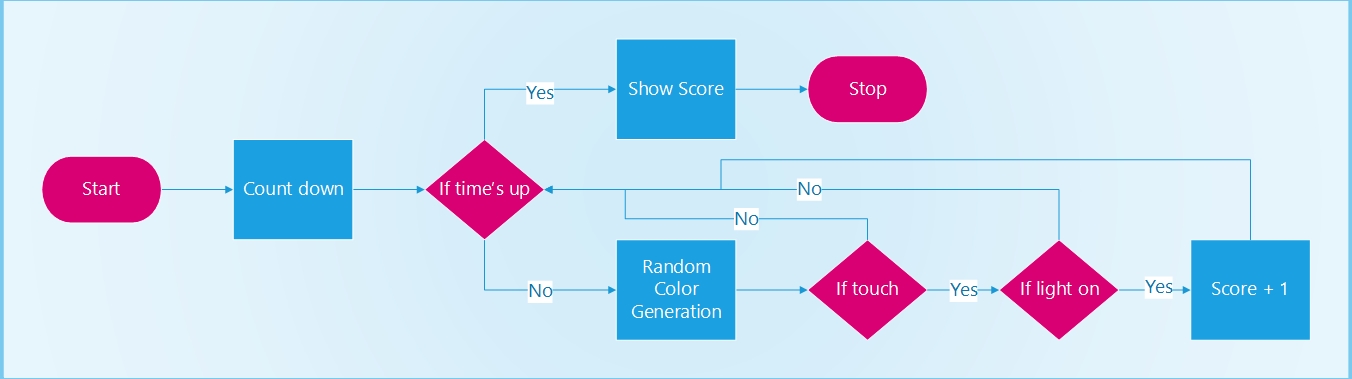
Example of Code
Import Module
from nanoleafapi import Nanoleaf, NanoleafDigitalTwin, RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE, WHITE
import random
import time
Defining Components
panel_id_list = nl.get_ids()
color_list = [RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE]
score = ""
second = ""
times_up = ""
Color Generation Function
Randomly pick a canvas and color from the lists.
def color_gen():
panel = random.choice(panel_id_list)
if digital_twin.get_color(panel) == (0,0,0):
digital_twin.set_color(panel, random.choice(color_list))
digital_twin.sync()
time.sleep(2)
Touch Function
Listen to the touch event.
Light off the panel when a touch event occurs and add the score.
event_dict = {}
def event_function(event):
print(event)
event_dict.update(event)
nl.register_event(event_function, [4])
def touch():
global score
global event_dict
try:
touch_id = event_dict['events'][0]['panelId']
if touch_id != -1:
try:
digital_twin.set_color(touch_id, (0,0,0))
event_dict['events'][0]['panelId'] = -1
score = score + 1
print("Score: " + str(score))
except:
pass
except KeyError:
pass
Score Display Function
Display the score by changing the color in the same amounts of canvas to light blue.
def score_display():
for i in panel_id_list(range(score)):
digital_twin.set_color(i, LIGHT_BLUE)
digital_twin.sync()
Countdown Function
Set the timer for 15 seconds.
def countdown():
second = second + 1
if second == 15:
times_up = 1
Game Initiation
score = 0
second = 0
times_up = 0
digital_twin.set_all_colors((0,0,0))
digital_twin.sync()
Game Structure
while True:
countdown()
time.sleep(1)
if times_up == 0:
touch()
color_gen()
if times_up == 1:
digital_twin.set_all_colors((0,0,0))
digital_twin.sync()
score_display()