Logic Flow
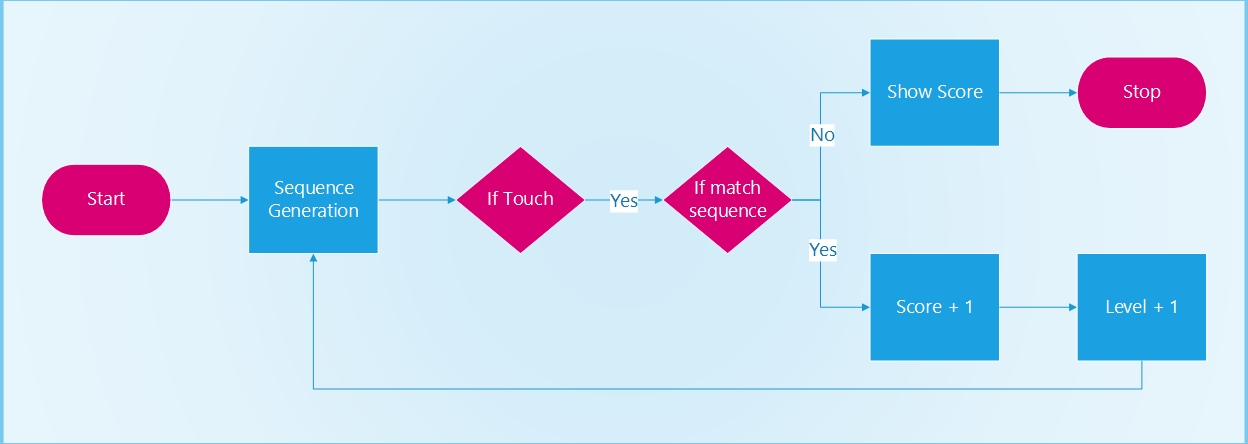
Example of Code
Import Module
from nanoleafapi import Nanoleaf, NanoleafDigitalTwin, RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE, WHITE
import random
import time
Defining Components
panel_id_list = nl.get_ids()
color_list = [RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE, WHITE]
sequence = {}
checklist = []
touch_wait = ""
score = ""
level = ""
end_game = ""
Sequence Generation Function
Randomly pick a block and append it into the sequence.
def sequence_gen():
sequence.update({len(sequence) + 1:[random.choice(panel_id_list), random.choice(color_list)]})
for i in sequence:
digital_twin.set_color(sequence[i][0],sequence[i][1])
digital_twin.sync()
time.sleep(3)
digital_twin.set_all_colors((0,0,0))
touch_wait = 1
Touch Event Listener and Touch Check Function
Listen to the touch event.
Check whether the touch event matches the order of the sequence.
event_dict = {}
def event_function(event):
print(event)
event_dict.update(event)
nl.register_event(event_function, [4])
def touch_check():
sequence_copy = sequence.copy()
for i in sequence_copy:
check_list.append((sequence_copy[i][0],sequence_copy[i][1])
touch_id = event_dict['events'][0]['panelId']
if touch_id == check_list[0]:
digital_twin.set_color(check_list[0][0],check_list[0][1])
del check_list[0]
if touch_id != check_list[0]:
end_game = 1
digital_twin.set_all_colors((0,0,0))
if len(check_list) == 0:
score = score + 1
level = level + 1
touch_wait = 0
Score Display Function
def score_display():
for i in panel_id_list(range(score)):
digital_twin.set_color(i, LIGHT_BLUE)
digital_twin.sync()
Game Initiation
score = 0
level = 1
touch_wait = 0
end_game = 0
Game Structure
while True:
if end_game == 0 and touch_wait == 0:
sequence_gen()
if touch_wait == 1:
touch_check()
if end_game == 1:
score_display()