Logic Flow
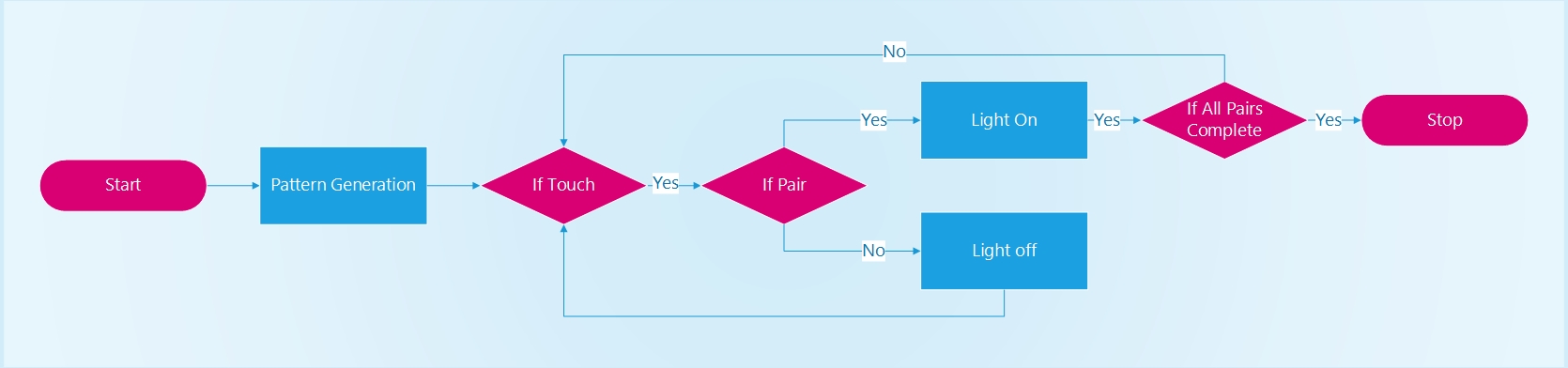
Example of Code
Import Module
from nanoleafapi import Nanoleaf, NanoleafDigitalTwin, RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE, WHITE
import random
import time
Defining Components
panel_id_list = nl.get_ids()
color_list = [RED, ORANGE, YELLOW, GREEN, LIGHT_BLUE, BLUE, PINK, PURPLE, WHITE]
color_pair = ""
color_pair_dict = {}
touch_check_list = []
end_game = ""
Pattern Generation Function
Generate memory pairs of patterns.
def pattern_gen():
panel_id_rand_list = panel_id_list.copy()
color_rand_list = color_list.copy()
random.shuffle(panel_id_rand_list)
random.shuffle(color_rand_list)
color_pair = 0
for i in panel_id_rand_list:
if color_pair < 2:
digital_twin.set_color(panel_id_rand_list[i],color_rand_list[0])
color_pair_dict.update({panel_id_rand_list[i]:color_rand_list[0]})
color_pair = color_pair + 1
del panel_id_rand_list[i]
if color_pair == 2:
del color_rand_list[0]
color_pair = 0
if len(color_rand_list) == 0:
color_rand_list = color_list.copy()
random.shuffle(color_rand_list)
if len(panel_id_rand_list) == 0:
digital_twin.sync()
time.sleep(5)
digital_twin.set_all_colors((0,0,0))
Touch Event Listener
Listen to the touch event.
event_dict = {}
def event_function(event):
print(event)
event_dict.update(event)
nl.register_event(event_function, [4])
Pair Check Function
Check whether the touch events occur in the same color of panels
def pair_check():
touch_id = event_dict['events'][0]['panelId']
digital_twin.set_color(touch_id,color_pair_dict[touch_id])
digital_twin.sync()
touch_check_list.append(touch_id)
if len(touch_check_list) == 2:
if digital_twin.get_color(touch_check_list[0]) == digital_twin.get_color(touch_check_list[1]):
for i in touch_check_list:
del color_pair_dict[i]
else:
for i in touch_check_list:
digital_twin.set_color(i,(0,0,0))
if len(color_pair_dict) == 0:
end_game = 1
Game Initiation
global color_pair
global color_pair_dict
global touch_check_list
end_game = 0
Game Structure
pattern_gen()
while True:
if end_game == 0:
pair_check()
if end_game == 1:
digital_twin.set_all_colors(WHITE)